Introduction
Unreal Engine 5 (UE5) is packed with tools that empower developers to create visually stunning and deeply engaging game mechanics. Among its most essential systems for developing complex gameplay is the Gameplay Ability System (GAS). At the core of this system are Gameplay Effect Components, which define how abilities and interactions impact game characters and objects.
Gameplay Effect Components are highly modular and customizable, allowing developers to build a wide range of effects, from buffs and debuffs to status changes like poison or stun. This guide breaks down what Gameplay Effect Components are, their key features, and how they can be used to create scalable, dynamic game mechanics.
What Are Gameplay Effect Components?
Gameplay Effect Components in Unreal Engine 5 are modular building blocks designed to enhance the behavior of Gameplay Effects within the Gameplay Ability System. They provide a flexible, data-driven way to implement complex interactions and effects on characters, objects, or the environment without the need for heavy coding.
Key Features of Gameplay Effect Components:
- Modularity: Components can be easily added or removed from gameplay effects, allowing for quick customization.
- Reusability: Once created, components can be reused across multiple gameplay effects, saving development time.
- Extensibility: Developers can create custom components to meet specific game mechanics.
- Performance Optimization: Designed to be efficient, scalable, and performant, even for large projects.
List of Gameplay Effect Components
Target Tags Component
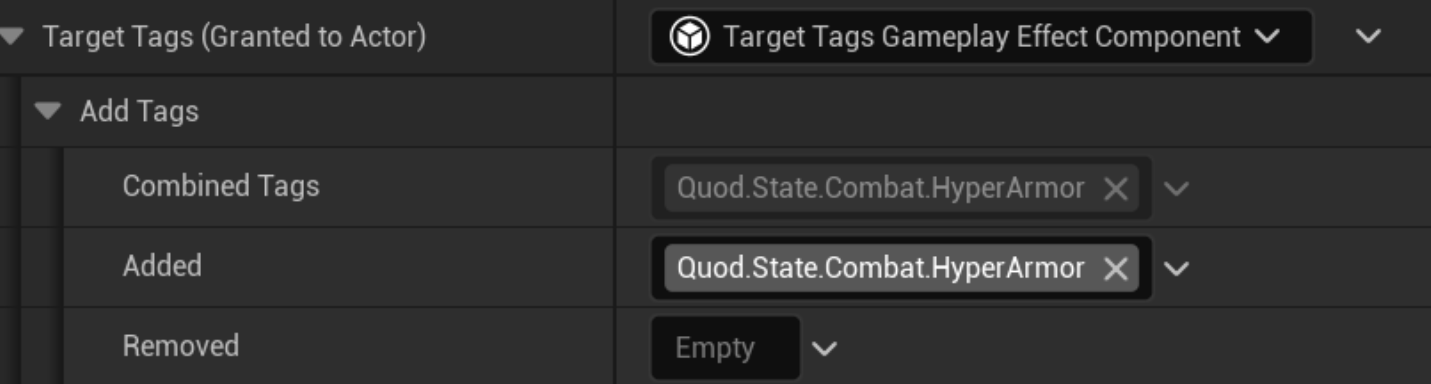
This component will grant the tags specified on it to the target of the effect. So if you apply an effect with this component to a character, that character will inherit all the tags that this effect component has configured.
You will see that in the Add Tags property you see a list of Combined, Added and Removed tags and feel like this is confusing. You will see this pattern used a lot in GAS when dealing with inheritance.
How it works is that what matters is the field Combined Tags. Then if you want to add a tag you put it in the Added field and the Combined field will auto update. If the Combined field already had some tags from the parent effect that you don't want simply add that to the Removed field and that tag will be gone.
Needing to handle inheritance this way is an unfortunate side effect of how Unreal tracks inherited properties, so you may need to get used to working this way.
In the end, in most cases you will simply add the tags you want in the Added field and be done with it.
Target Tag Requirements Component
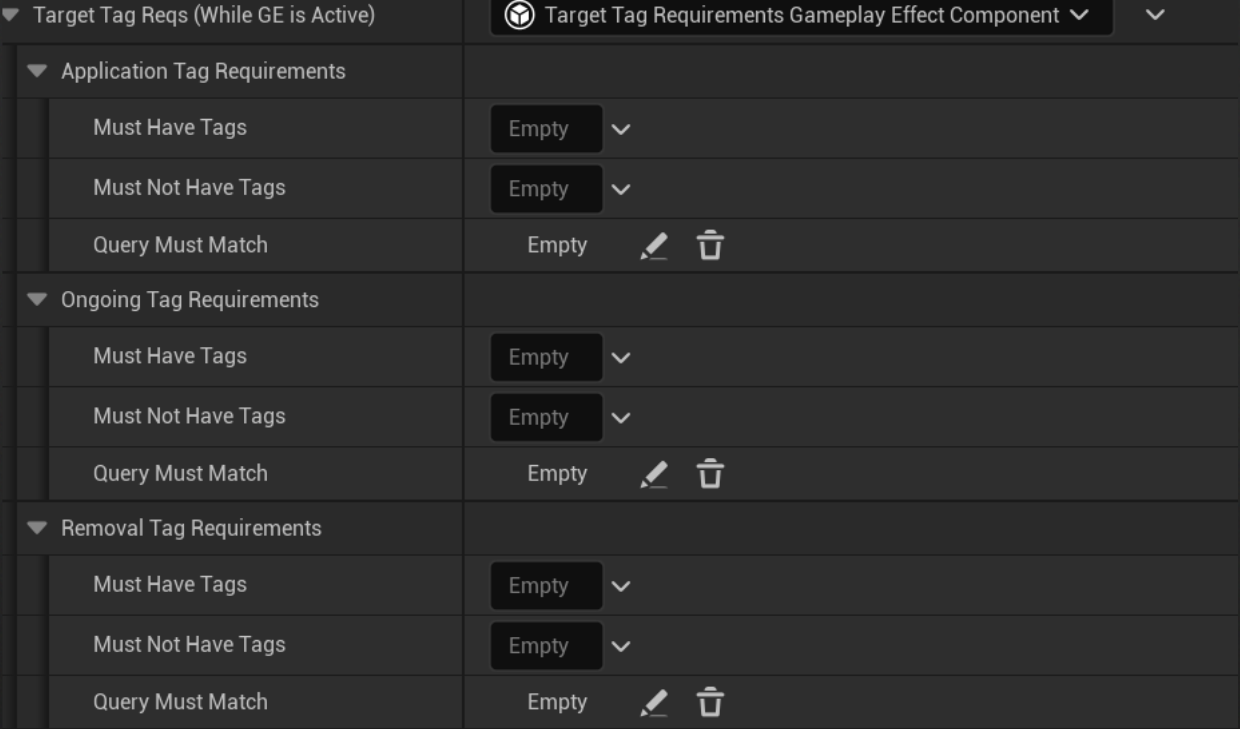
This component will check the presence of tags on a target to verify if the effect can be applied or not. It can also be used to activate or deactivate the effect based on tags that the target may have as or remove the effect if certain tag conditions are met.
Application Tag Requirements
This will be checked the moment the effect is applied.
For the application to succeed all tags in Must Have Tags have to be on the target and NONE of the tags in Must not Have Tags have not to be present on the target.
Finally, the Query Must Match query property needs to be true. This query is optional, but allows to configure more complex logic like certain tags A being present OR certain tag B being present but not BOTH being present at the same time.
Ongoing Tag Requirements
This works similarly to the Application Tags but will be an ongoing check. If the conditions are fulfilled the effect will be activated and if the conditions are not met the effect will be disabled.
Disabled effects do not apply their modifiers and certain Effect Components may behave differently based on the effect being active or not.
It only makes sense for HasDuration or Infinite effects.
Removal Tag Requirements
Similar to the Application and Ongoing categories this will check conditions to see if the effect has to be removed.
Quite useful to dinamically remove effects the moment certain tags are present, or to remove buffs the moment other tags are removed from the target. Like linking an "Extra Damage" effect being on the character only until the "State.Combat.Rage" tag is also present.
This category also only makes sense for HasDuration and Infinite effects.
Chance to Apply Component

If this component exist on the effect, the moment the effect is going to be applied a random chance check will be executed and the effect will only be applied if that "dice roll" succeeds.
The chance to apply can be configured with a float or via a CurveTable based on the effect level.
( Effect Level can be configured the moment you apply the effect )
Grant Abilities Component
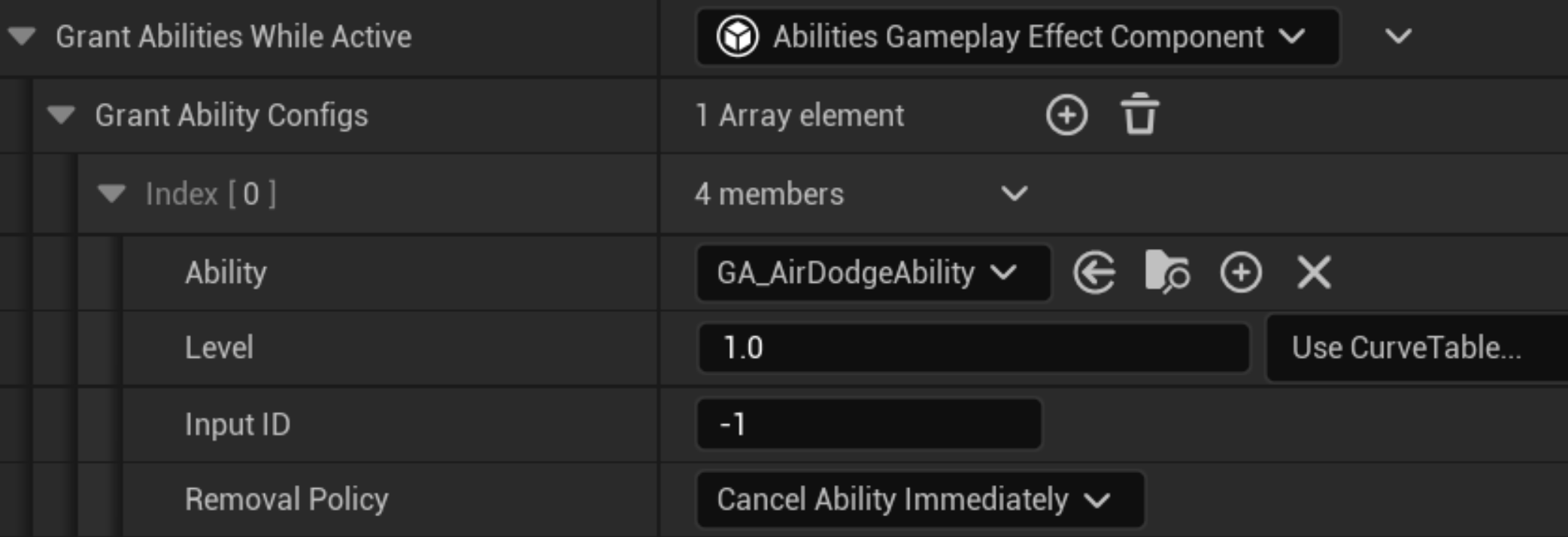
Grant Abilities Component allows you to Grant Abilities to the target of the effect while that effect is applied to the target.
This is incredibly useful, as you could create a trigger box that applies a "Double Jump Effect" that simply grants the double jump ability, and suddenly you quickly have a "Double Jump Area" implemented in your game.
You can also decide how the ability is removed, like canceling the moment the effect is removed or waiting for the ability to end naturally after removing the effect.
Additional Effects Component
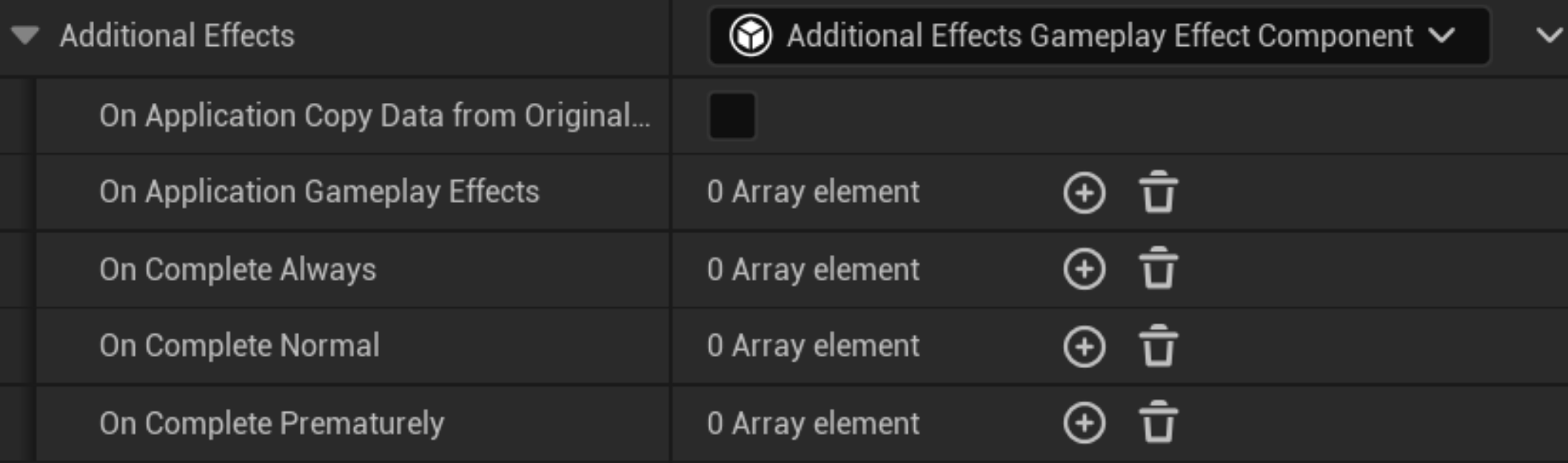
This component will allow you to apply additional effects at certain points of the effect lifecycle.
If you want to apply some extra effects the moment the effect is applied then put the m on the On Application Gameplay Effects property.
For applying extra effects when the effect completes you have three options:
- On Complete Always: As the name implies, the moment the effect is removed this extra effects will be applied
- On Complete Normal: Usually for effects that have duration, when the duration ends these effects will be applied
- On Complete Prematurely: If the effect still had some duration but you remove it or it gets cancelled due to other components like the Target Tag Requirements Component then these effects will be applied instead of the On Complete Normal
By the way, I always recommend checking the On Application Copy Data From Original Spec checkbox, as that way your extra effects will receive all the Set By Caller values from the original effect.
Asset Tags Component
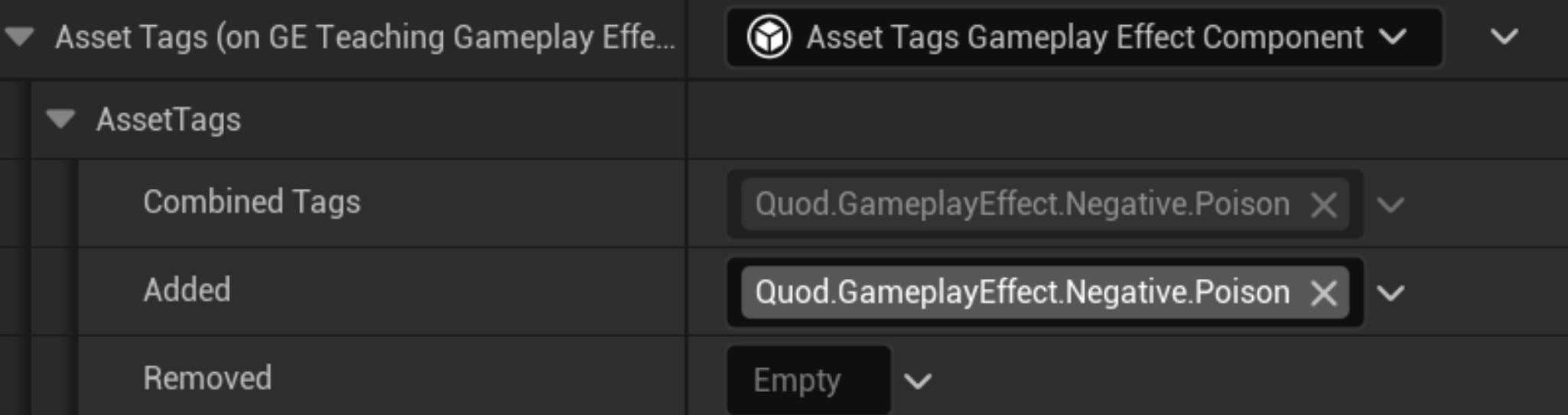
The same way you can apply tags to the target of the effect, the effect itself can have tags.
Why is this useful you ask?
This way you will be able to query for effects tagged in certain ways, and as you will see a little further down other components will use this tags to apply or not their behavior.
Immunity Component

Immunity allows you to block the application of other effects. You c an add multiple immunity queries.
This allows you to check multiple things, so let's go one by one:
- Owning Tag Query: Check tags that the effect being applied grants to the character
- Effect Tag Query: Check tags that the effect being applied has
- Source Spec Tag Query: This is checked against the spec captured tags of the source
- Modifying Attribute: If the effect being applied modifies this attribute, then the immunity query will prevent application
- Source Aggregate Tag Query: This checks against the tags that the source of this effect has ( The source could be a weapon for instance, then tags on the weapon would be checked )
- Effect Source: If the Source Object that is applying this effect is the one selected here, the immunity query will be true
- Effect Definition: Allows you to check for a specific class of Gameplay Effect for the immunity query
As you can see, there are a lot of things you can configure here and a lot of them require deep understanding of how GAS works and applies effects.
My recommendation is that in most cases you simply use the Effect Tag Query to check tags on the effects.
For instance if you create a poison effect, you can tag it as Effect.Negative.Poison and then when you equip the armor that grants immunity to poison, simply apply an Infinite effect with an immunity query checking for that Effect.Negative.Poison tag on the Effect Tag Query.
Remove Other Effects Component
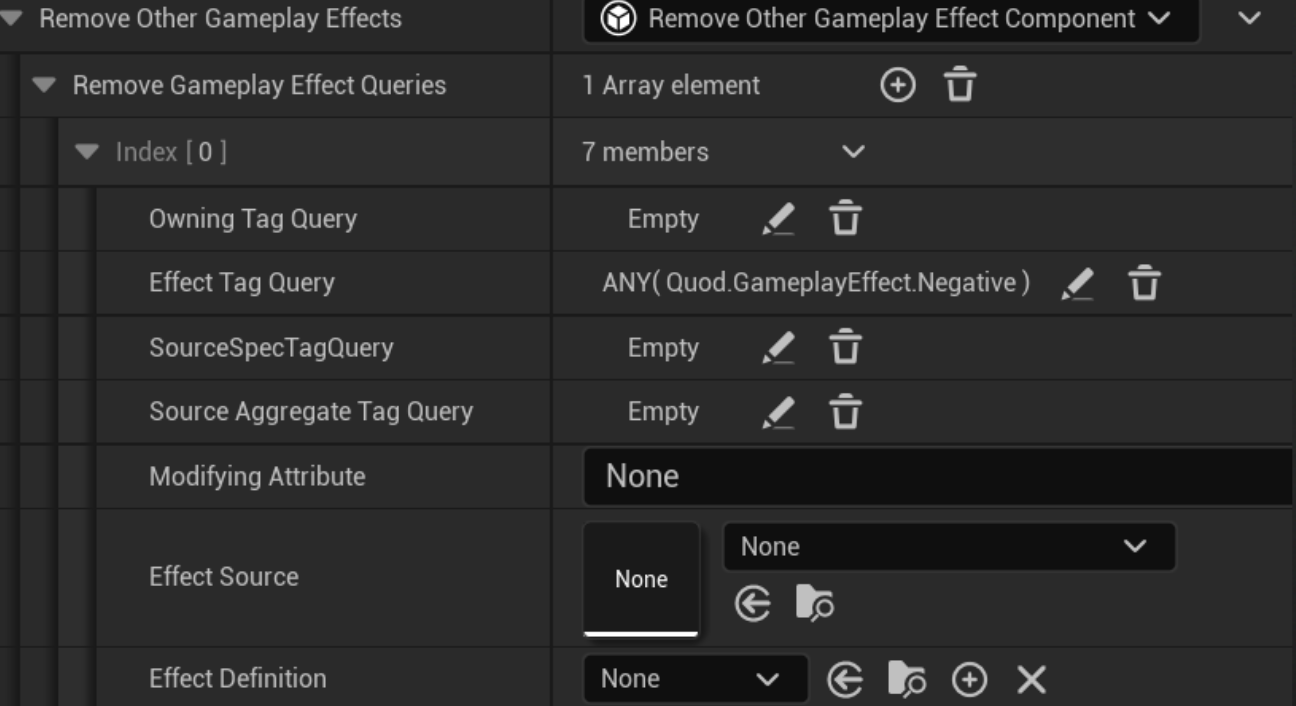
The same way you can become immune to the application of certain effects, you can also remove other effects when a Gameplay Effect is applied.
The configurable properties are the same ones as the previous component.
Following the poison example from before, you could implement an item in an RPG Game that cures all status effects by adding a Remove Other Effects Component with the Effect Tag Query for the tag Effect.Negative.
If you negative effects have that tag ( remember that Gameplay Tags are hierarchical, so Effect.Negative.Poison matches the Effect.Negative query ) then all negative effects will be removed the moment you apply this effect.
Block Ability Tags Component
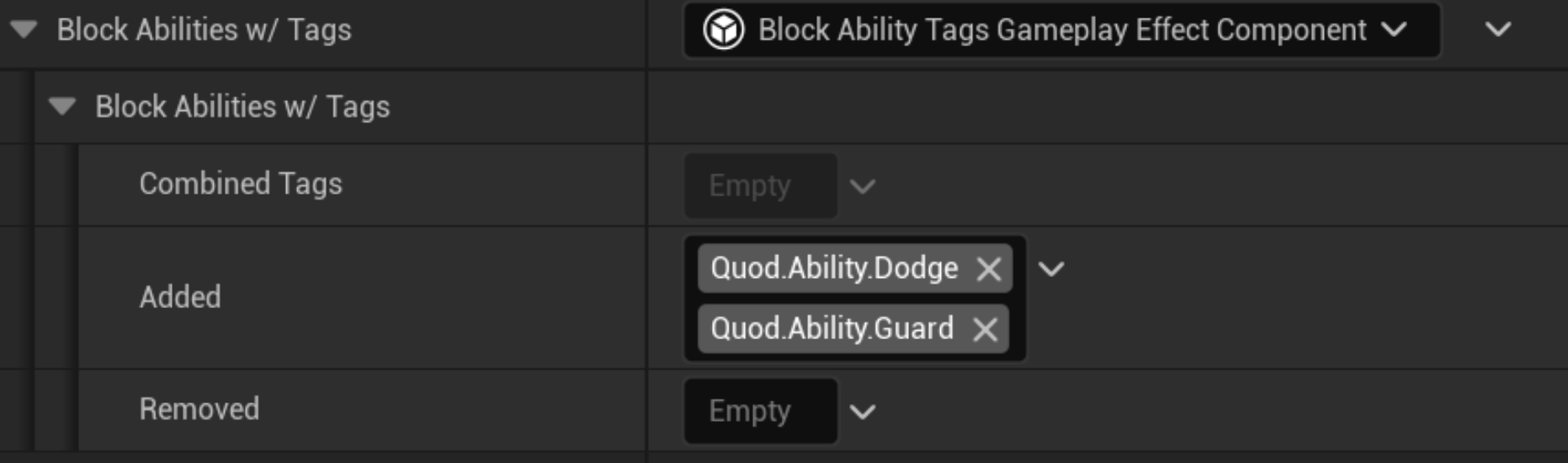
The same way that effects can have tags, Gameplay Abilities can also have tags.
This components block abilities with the configured tags and prevent their activation.
If you want a spell that applies a "Prevent Cast Magic" debuff you can simply create a HasDuration effect with a 10s duration and a Block Ability Tags Component that will block all abilities tagged with Ability.Magic.
As always, abilities need to be properly tagged for this to work properly.
Custom Can Apply Component

The same way that you have some built-in tags queries to check if an effect can be applied, this component allows you to write custom code to check things that may be project-specific.
For instance, you could write a Custom Application Requirement that requires the target of the effect to be of a certain faction, or be targeted by at least 3 enemies or whatever other custom logic you want.
So if none of the other requirement components work for you, before trying to implement a custom component you can implement a Custom Application Requirement.
UI Data Component

This component allows you to link UI data to the effect.
By default it only supports a description, but the ideal way to use this is to inherit from this component and configure it with any custom data you need.
For instance you can inherit and add data for an icon and then iterate all applied effects that have UI Data and display all the icons under the health bar of the character.
Conclusion
Gameplay Effect Components in Unreal Engine 5 are powerful tools for creating highly modular, reusable, and customizable game mechanics. By utilizing these components, developers can manage complex effects efficiently, saving time and reducing the need for extensive coding.
Mastering Gameplay Effect Components requires practice and experimentation. Start by implementing simple components and progressively introduce more advanced logic. Whether you're building an RPG with intricate status effects or an action game with dynamic abilities, these components provide a solid foundation for flexible and scalable gameplay design.
As you refine your approach, don’t hesitate to push the boundaries of what’s possible. With Gameplay Effect Components, you have the tools to create rich, interactive worlds that respond to the player’s actions in meaningful ways.
I hope this article has helped you understand the Gameplay Ability System a little bit more!